How Developers Can Enhance Their Debugging Competencies By Gustavo Woltmann
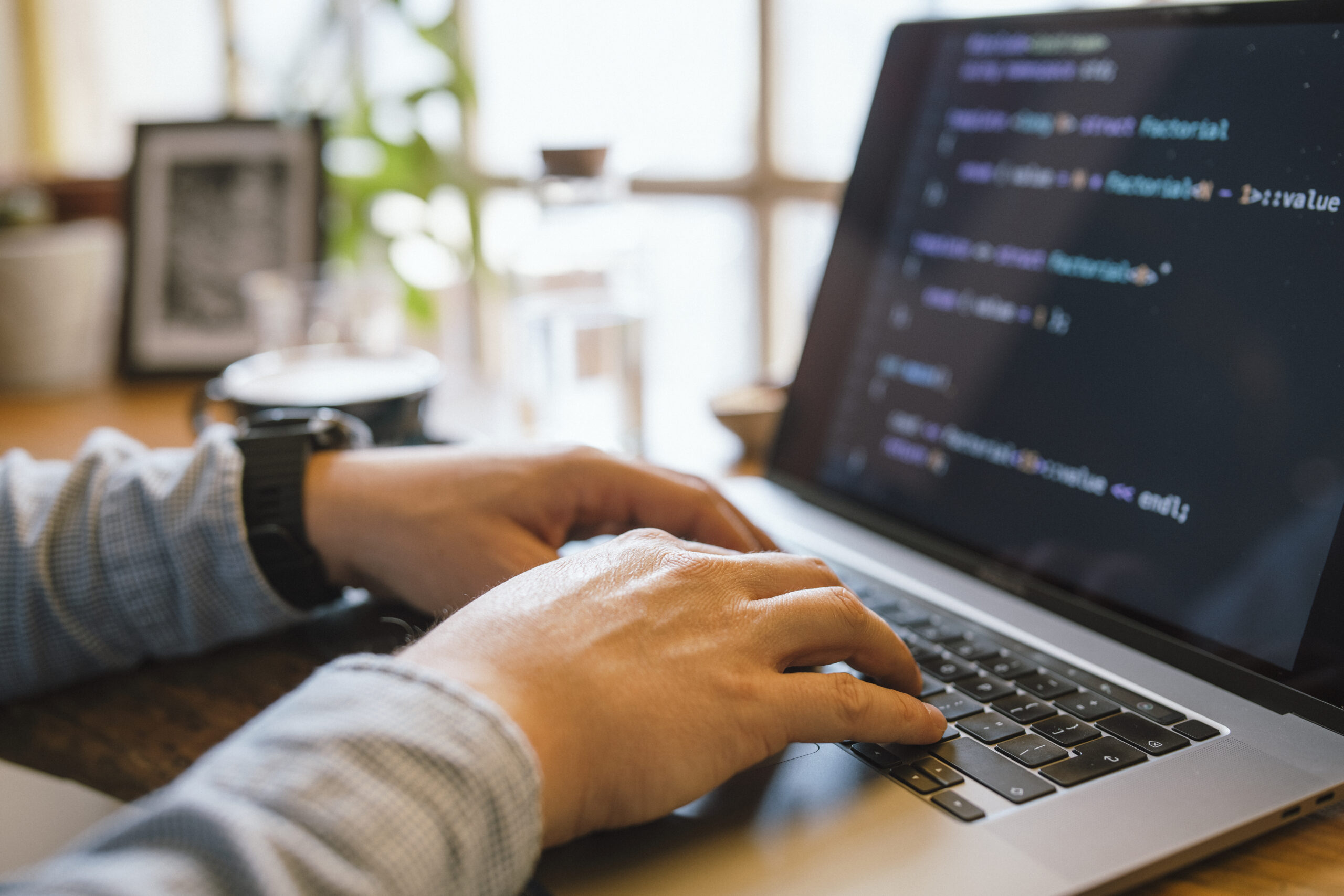
Debugging is one of the most essential — nevertheless generally missed — abilities within a developer’s toolkit. It's actually not pretty much correcting damaged code; it’s about understanding how and why items go Completely wrong, and Mastering to Believe methodically to resolve difficulties successfully. Whether you're a beginner or perhaps a seasoned developer, sharpening your debugging abilities can conserve hours of aggravation and drastically boost your productivity. Listed here are numerous techniques to aid developers level up their debugging game by me, Gustavo Woltmann.
Learn Your Applications
On the list of quickest approaches builders can elevate their debugging techniques is by mastering the instruments they use on a daily basis. Though crafting code is just one Component of progress, being aware of the way to communicate with it efficiently in the course of execution is equally important. Contemporary improvement environments come equipped with strong debugging capabilities — but many builders only scratch the floor of what these resources can do.
Get, such as, an Built-in Progress Natural environment (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These equipment let you set breakpoints, inspect the worth of variables at runtime, action by code line by line, and in some cases modify code about the fly. When utilized effectively, they Enable you to observe exactly how your code behaves all through execution, which happens to be a must have for tracking down elusive bugs.
Browser developer applications, for instance Chrome DevTools, are indispensable for front-finish builders. They assist you to inspect the DOM, watch network requests, see genuine-time performance metrics, and debug JavaScript from the browser. Mastering the console, sources, and community tabs can turn discouraging UI problems into workable duties.
For backend or method-amount developers, applications like GDB (GNU Debugger), Valgrind, or LLDB offer you deep Handle more than functioning processes and memory administration. Studying these applications might have a steeper Discovering curve but pays off when debugging functionality issues, memory leaks, or segmentation faults.
Further than your IDE or debugger, turn into snug with Model control techniques like Git to be aware of code history, locate the precise minute bugs were launched, and isolate problematic variations.
In the end, mastering your applications means heading further than default settings and shortcuts — it’s about producing an personal knowledge of your growth ecosystem to ensure that when troubles occur, you’re not shed in the dark. The higher you understand your instruments, the more time you are able to commit resolving the particular trouble as opposed to fumbling through the method.
Reproduce the trouble
One of the most vital — and sometimes ignored — actions in successful debugging is reproducing the situation. Right before leaping into the code or producing guesses, developers need to have to produce a consistent surroundings or circumstance exactly where the bug reliably appears. With no reproducibility, correcting a bug gets to be a recreation of prospect, generally bringing about wasted time and fragile code changes.
The initial step in reproducing a dilemma is accumulating just as much context as possible. Talk to queries like: What steps resulted in The difficulty? Which natural environment was it in — advancement, staging, or creation? Are there any logs, screenshots, or error messages? The greater detail you have got, the a lot easier it becomes to isolate the precise ailments underneath which the bug happens.
Once you’ve collected enough information, endeavor to recreate the trouble in your neighborhood setting. This could mean inputting exactly the same data, simulating identical consumer interactions, or mimicking system states. If The problem seems intermittently, look at creating automatic tests that replicate the edge conditions or condition transitions included. These exams don't just support expose the issue but will also avert regressions Down the road.
Often, the issue can be surroundings-certain — it'd come about only on specified functioning methods, browsers, or below individual configurations. Making use of instruments like virtual devices, containerization (e.g., Docker), or cross-browser testing platforms might be instrumental in replicating such bugs.
Reproducing the challenge isn’t simply a stage — it’s a mentality. It demands endurance, observation, in addition to a methodical method. But after you can continually recreate the bug, you might be already halfway to repairing it. By using a reproducible state of affairs, You should use your debugging equipment additional effectively, test likely fixes safely and securely, and connect additional Evidently with your team or customers. It turns an abstract complaint into a concrete challenge — Which’s where by builders prosper.
Examine and Have an understanding of the Mistake Messages
Mistake messages will often be the most beneficial clues a developer has when a little something goes wrong. As opposed to viewing them as irritating interruptions, developers ought to learn to take care of mistake messages as immediate communications from your program. They generally inform you just what exactly transpired, the place it took place, and from time to time even why it transpired — if you know the way to interpret them.
Start by reading the message diligently As well as in entire. A lot of developers, specially when less than time force, look at the primary line and quickly start off generating assumptions. But deeper during the mistake stack or logs could lie the accurate root cause. Don’t just duplicate and paste error messages into engines like google — study and realize them initial.
Crack the error down into components. Can it be a syntax error, a runtime exception, or a logic error? Does it issue to a certain file and line variety? What module or perform triggered it? These issues can manual your investigation and issue you toward the responsible code.
It’s also valuable to be aware of the terminology in the programming language or framework you’re applying. Error messages in languages like Python, JavaScript, or Java often comply with predictable patterns, and Finding out to acknowledge these can dramatically increase your debugging method.
Some faults are obscure or generic, and in People instances, it’s essential to look at the context by which the mistake occurred. Test connected log entries, enter values, and recent alterations during the codebase.
Don’t neglect compiler or linter warnings possibly. These typically precede more substantial challenges and provide hints about opportunity bugs.
In the long run, error messages are not your enemies—they’re your guides. Understanding to interpret them effectively turns chaos into clarity, encouraging you pinpoint problems more rapidly, reduce debugging time, and become a far more successful and self-assured developer.
Use Logging Wisely
Logging is Probably the most strong instruments inside of a developer’s debugging toolkit. When used successfully, it provides true-time insights into how an software behaves, encouraging you comprehend what’s taking place beneath the hood while not having to pause execution or move in the code line by line.
A good logging technique begins with being aware of what to log and at what stage. Widespread logging degrees include things like DEBUG, Facts, Alert, ERROR, and Lethal. Use DEBUG for specific diagnostic information and facts during improvement, INFO for general events (like successful start-ups), Alert for probable difficulties that don’t split the appliance, ERROR for actual problems, and Lethal if the program can’t proceed.
Keep away from flooding your logs with too much or irrelevant details. Too much logging can obscure critical messages and decelerate your program. Give attention to important events, point out adjustments, input/output values, and critical decision details within your code.
Structure your log messages clearly and continuously. Include context, such as timestamps, request IDs, and performance names, so it’s much easier to trace issues in dispersed systems or multi-threaded environments. Structured logging (e.g., JSON logs) might make it even simpler to parse and filter logs programmatically.
For the duration of debugging, logs Enable you to monitor how variables evolve, what situations are met, and what branches of logic are executed—all without the need of halting the program. They’re Particularly precious in manufacturing environments where by stepping by means of code isn’t probable.
Moreover, use logging frameworks and tools (like Log4j, Winston, or Python’s logging module) that assist log rotation, filtering, and integration with checking dashboards.
In the end, intelligent logging is about stability and clarity. That has a nicely-imagined-out logging approach, it is possible to reduce the time it will take to identify issues, achieve further visibility into your purposes, and improve the Total maintainability and trustworthiness of one's code.
Assume Like a Detective
Debugging is not only a complex endeavor—it's a kind of investigation. To correctly determine and resolve bugs, builders should technique the procedure similar to a detective resolving a mystery. This attitude can help stop working complicated troubles into workable pieces and follow clues logically to uncover the root trigger.
Commence by collecting proof. Think about the symptoms of the issue: error messages, incorrect output, or efficiency troubles. Identical to a detective surveys against the law scene, obtain just as much relevant information as you are able to without having jumping to conclusions. Use logs, examination circumstances, and consumer studies to piece collectively a clear image of what’s taking place.
Upcoming, sort hypotheses. Talk to yourself: What might be creating this behavior? Have any modifications lately been produced to the codebase? Has this issue occurred before under equivalent conditions? The purpose is usually to narrow down choices and recognize prospective culprits.
Then, examination your theories systematically. Try and recreate the situation in the controlled atmosphere. In the event you suspect a specific functionality or ingredient, isolate it and confirm if the issue persists. Similar to a detective conducting interviews, check with your code thoughts and Permit the final results direct you closer to the truth.
Pay shut interest to small aspects. Bugs generally cover within the least expected sites—like a lacking semicolon, an off-by-a single mistake, or maybe a race issue. Be comprehensive and affected individual, resisting the urge to patch The problem without having totally comprehending it. Momentary fixes might disguise the real challenge, only for it to resurface later on.
Lastly, retain notes on Everything you tried using and discovered. Equally as detectives log their investigations, documenting your debugging method can help you save time for potential difficulties and assist Some others realize your reasoning.
By imagining like a detective, developers can sharpen their analytical competencies, method challenges methodically, and turn out to be more practical at uncovering hidden troubles in elaborate methods.
Publish Checks
Creating assessments is among the simplest ways to boost your debugging techniques and In general development efficiency. Exams not simply assistance catch bugs early but additionally serve as a safety Internet that offers you confidence when producing variations to your codebase. Developers blog A nicely-examined software is simpler to debug as it helps you to pinpoint exactly exactly where and when an issue occurs.
Start with unit tests, which concentrate on personal features or modules. These smaller, isolated checks can immediately expose regardless of whether a particular piece of logic is Functioning as anticipated. Whenever a check fails, you instantly know in which to search, considerably decreasing the time used debugging. Device assessments are In particular valuable for catching regression bugs—troubles that reappear right after Earlier getting set.
Subsequent, combine integration assessments and conclude-to-stop tests into your workflow. These help make sure several portions of your application work alongside one another efficiently. They’re significantly valuable for catching bugs that take place in complicated systems with many elements or services interacting. If a little something breaks, your assessments can tell you which Component of the pipeline failed and under what ailments.
Composing tests also forces you to definitely Consider critically about your code. To check a attribute correctly, you need to be aware of its inputs, anticipated outputs, and edge conditions. This degree of being familiar with In a natural way leads to higher code composition and fewer bugs.
When debugging a difficulty, creating a failing take a look at that reproduces the bug may be a strong starting point. After the take a look at fails regularly, you may focus on repairing the bug and check out your test move when The difficulty is resolved. This strategy makes certain that exactly the same bug doesn’t return Sooner or later.
To put it briefly, writing assessments turns debugging from the irritating guessing match right into a structured and predictable process—aiding you capture extra bugs, faster and even more reliably.
Acquire Breaks
When debugging a tough issue, it’s simple to become immersed in the situation—gazing your display for hrs, striving Option just after Answer. But The most underrated debugging instruments is solely stepping absent. Having breaks helps you reset your thoughts, minimize stress, and sometimes see The problem from a new viewpoint.
When you are way too near to the code for way too prolonged, cognitive tiredness sets in. You would possibly start out overlooking noticeable faults or misreading code you wrote just several hours previously. In this particular condition, your brain turns into significantly less effective at issue-solving. A brief wander, a coffee break, or perhaps switching to a different task for ten–quarter-hour can refresh your emphasis. Several developers report finding the foundation of a challenge once they've taken time to disconnect, permitting their subconscious get the job done from the qualifications.
Breaks also aid stop burnout, Particularly during extended debugging periods. Sitting before a display, mentally stuck, is not simply unproductive but in addition draining. Stepping away helps you to return with renewed Power in addition to a clearer way of thinking. You could all of a sudden see a missing semicolon, a logic flaw, or a misplaced variable that eluded you in advance of.
In case you’re stuck, a superb rule of thumb should be to set a timer—debug actively for forty five–60 minutes, then have a 5–ten minute split. Use that point to move around, stretch, or do something unrelated to code. It could feel counterintuitive, In particular under restricted deadlines, but it essentially leads to speedier and more effective debugging Eventually.
In short, using breaks is just not an indication of weakness—it’s a wise strategy. It provides your Mind space to breathe, enhances your point of view, and helps you stay away from the tunnel eyesight That always blocks your progress. Debugging can be a mental puzzle, and rest is a component of resolving it.
Learn From Every single Bug
Each individual bug you experience is much more than simply A short lived setback—it's a chance to mature as being a developer. No matter whether it’s a syntax mistake, a logic flaw, or a deep architectural concern, each can instruct you something beneficial in case you make the effort to replicate and analyze what went Incorrect.
Commence by asking by yourself a handful of vital questions after the bug is settled: What triggered it? Why did it go unnoticed? Could it are already caught previously with superior tactics like device tests, code assessments, or logging? The responses normally expose blind places as part of your workflow or being familiar with and help you build stronger coding habits moving forward.
Documenting bugs can also be an excellent habit. Continue to keep a developer journal or maintain a log in which you Take note down bugs you’ve encountered, the way you solved them, and Whatever you uncovered. With time, you’ll start to see patterns—recurring challenges or popular faults—you can proactively keep away from.
In group environments, sharing Everything you've learned from a bug with all your friends could be Specifically potent. Whether it’s via a Slack concept, a short write-up, or A fast expertise-sharing session, assisting others steer clear of the identical issue boosts workforce effectiveness and cultivates a stronger Mastering tradition.
More importantly, viewing bugs as classes shifts your state of mind from irritation to curiosity. As an alternative to dreading bugs, you’ll begin appreciating them as critical areas of your development journey. In spite of everything, a number of the best developers are not the ones who generate best code, but those who repeatedly learn from their problems.
In the end, Every single bug you fix adds a completely new layer in your ability established. So up coming time you squash a bug, have a moment to mirror—you’ll occur away a smarter, far more able developer due to it.
Conclusion
Strengthening your debugging competencies requires time, exercise, and tolerance — however the payoff is big. It will make you a more effective, self-confident, and able developer. The next time you are knee-deep in the mysterious bug, try to remember: debugging isn’t a chore — it’s an opportunity to become far better at That which you do.